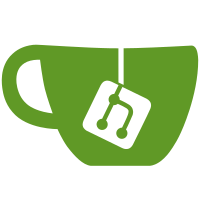
doublesided switch in blender 2.9x did not work before, it was always rendered single sided in MG. I added a new switch only-selectable which allows to create unvisible but selectable meshes. This helps with selection of small units which can now have an unvisible hand craftet select object around them for example..
77 lines
1.8 KiB
C++
77 lines
1.8 KiB
C++
// ==============================================================
|
|
// This file is part of Glest Shared Library (www.glest.org)
|
|
//
|
|
// Copyright (C) 2001-2008 Martiño Figueroa
|
|
//
|
|
// You can redistribute this code and/or modify it under
|
|
// the terms of the GNU General Public License as published
|
|
// by the Free Software Foundation; either version 2 of the
|
|
// License, or (at your option) any later version
|
|
// ==============================================================
|
|
|
|
|
|
#ifndef _SHARED_GRAPHICS_MODELRENDERER_H_
|
|
#define _SHARED_GRAPHICS_MODELRENDERER_H_
|
|
|
|
#include "model.h"
|
|
#include "leak_dumper.h"
|
|
|
|
namespace Shared{ namespace Graphics{
|
|
|
|
enum RenderMode{
|
|
rmNormal,
|
|
rmSelection,
|
|
rmShadows,
|
|
|
|
renderModeCount
|
|
};
|
|
|
|
|
|
class Texture;
|
|
|
|
// =====================================================
|
|
// class MeshCallback
|
|
//
|
|
/// This gets called before rendering mesh
|
|
// =====================================================
|
|
|
|
class MeshCallback{
|
|
public:
|
|
virtual ~MeshCallback(){};
|
|
virtual void execute(const Mesh *mesh)= 0;
|
|
};
|
|
|
|
// =====================================================
|
|
// class ModelRenderer
|
|
// =====================================================
|
|
|
|
class ModelRenderer{
|
|
protected:
|
|
bool renderNormals;
|
|
bool renderTextures;
|
|
bool renderColors;
|
|
bool colorPickingMode;
|
|
MeshCallback *meshCallback;
|
|
|
|
public:
|
|
ModelRenderer() {
|
|
renderNormals = false;
|
|
renderTextures = false;
|
|
renderColors = false;
|
|
colorPickingMode = false;
|
|
|
|
meshCallback= NULL;
|
|
}
|
|
|
|
virtual ~ModelRenderer(){};
|
|
|
|
virtual void begin(bool renderNormals, bool renderTextures, bool renderColors, bool colorPickingMode, MeshCallback *meshCallback= NULL)=0;
|
|
virtual void end()=0;
|
|
virtual void render(Model *model,int renderMode=rmNormal)=0;
|
|
virtual void renderNormalsOnly(Model *model)=0;
|
|
};
|
|
|
|
}}//end namespace
|
|
|
|
#endif
|